JavaFX: custom ListCell
Posted on July 3, 2013
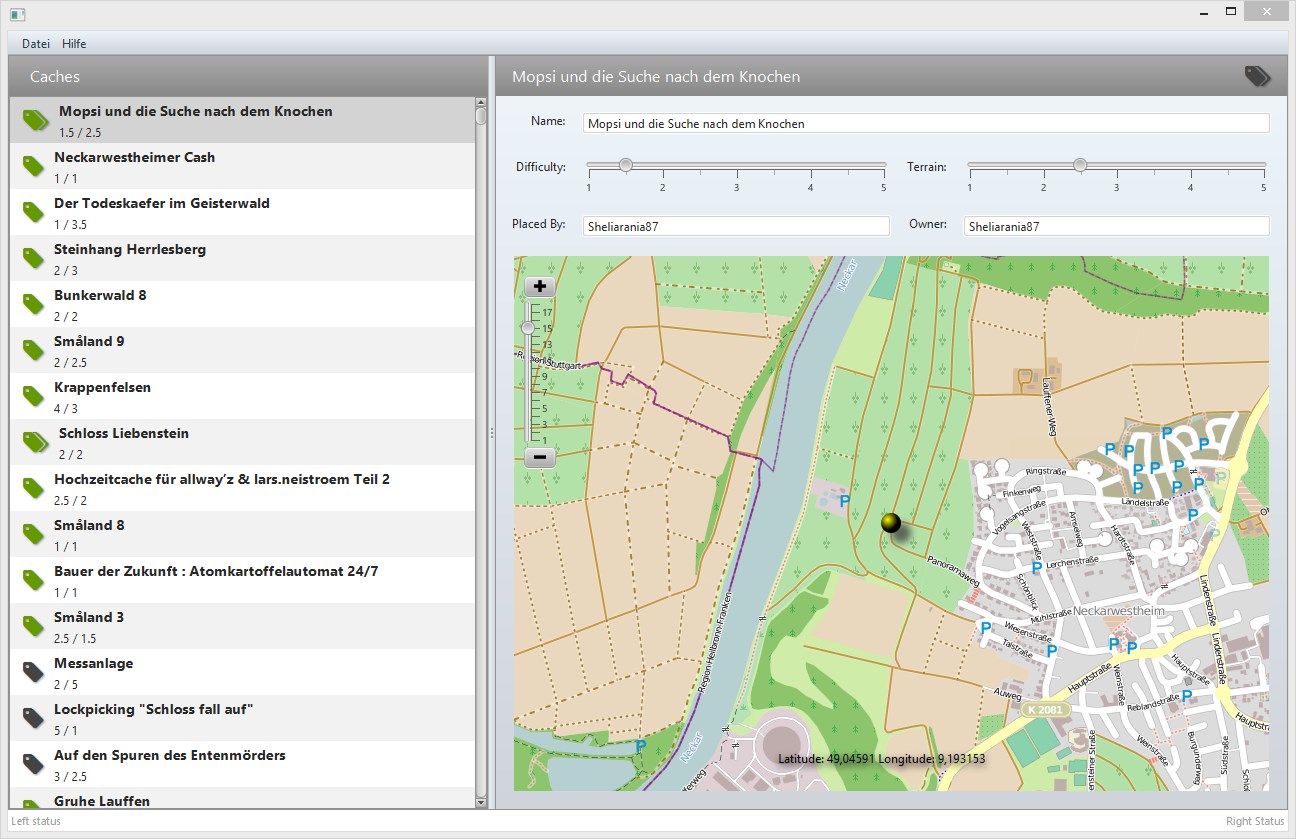
This post shows the change of a simple list to a multiline list with icons.
I have a list of geocaches in my GeoFroggerFX (replacement for my GeoCachingFrogger based on Netbeans) which should provide more information in a row.
Simple List
The first and simplest version had some textual rows.
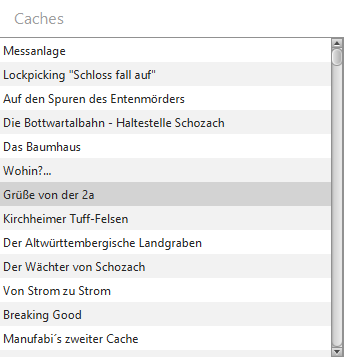
simple list
Custom CellList with more information
But this isn´t too sexy and I also wanted more information in my list. So I decided to use a custom ListCell to support more information in multiple rows and also some icons. The first version just added more information as text to the row.
|
|
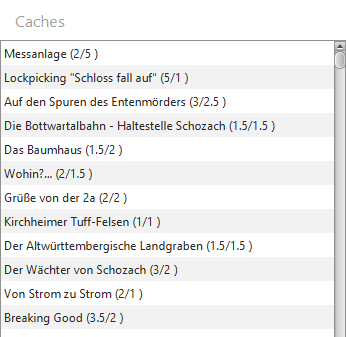
list with more information
Custom CellList with multiple columns and icon
The second version splits the information in multiple rows and adds an icon to the cell. I use FontAwesome as icon and not an image.
|
|
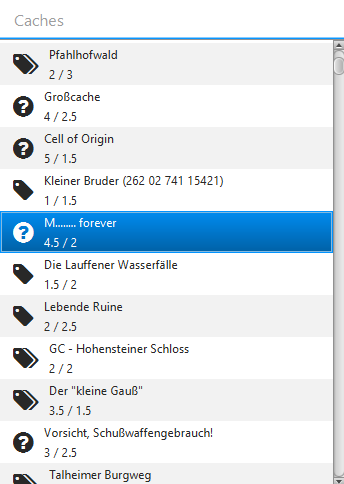
list with icons
Styling ListCell with css
I added some classes to the nodes to style the list with those classes.
|
|
This brings some colour into the list and the name of the cache is bigger than the rest.
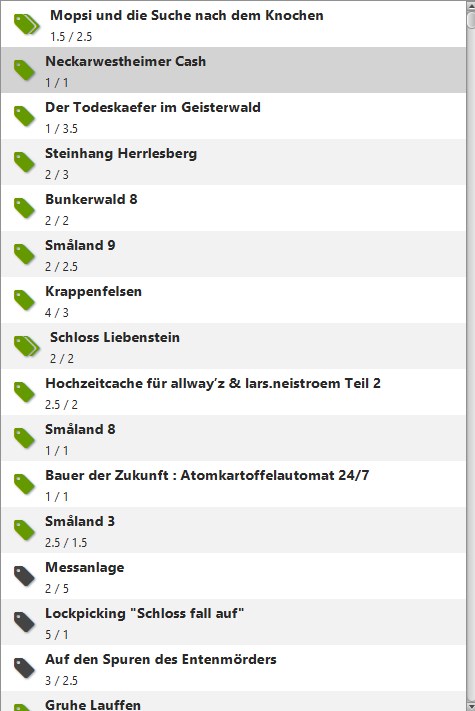
listcell with color
Jonathans tip how to do it THE RIGHT WAY
Jonathan Giles wrote me on twitter:
@frosch95 You shouldn’t create new instances of nodes in the updateItem method - instantiate them once in the cell constructor and reuse
So I refactored the class to reuse the objects and for better readability.
|
|