JavaFX: MiniIcon(Animation)Button refactored
Posted on August 22, 2012
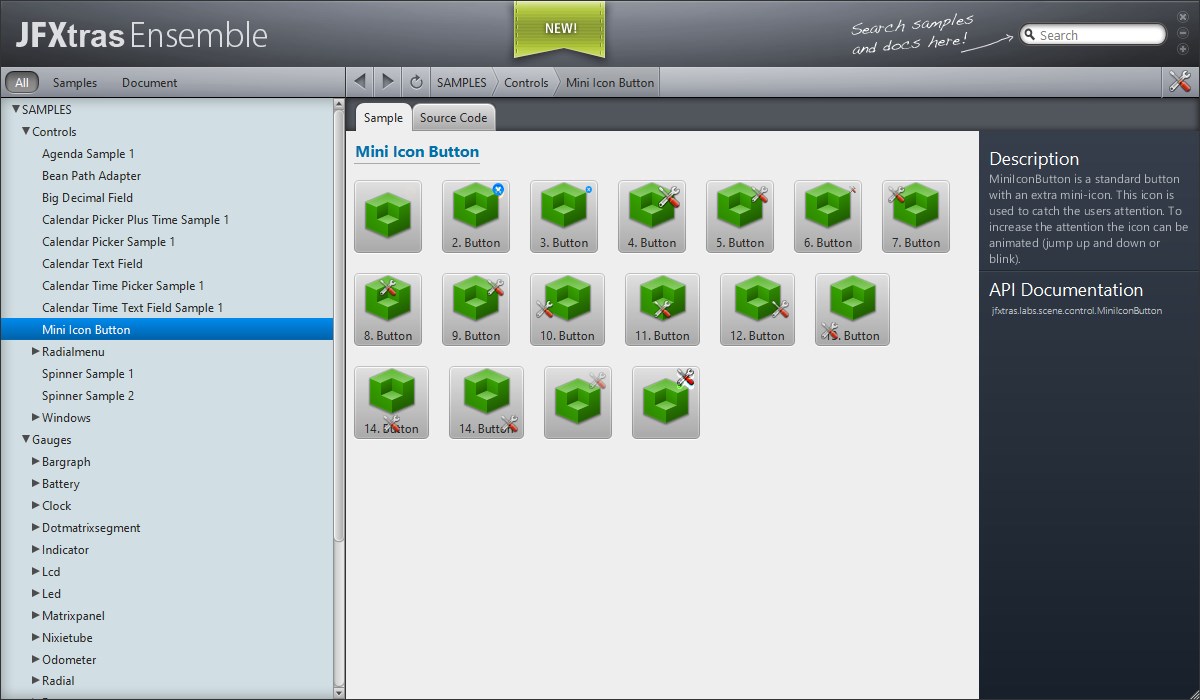
Refactored the control to add it to the JFXtras project.
Jonathan Giles wrote in his Blog that
This kind of code should be polished and added to the JFXtras project!
So I thought this would be a good start to learn more about JavaFX internals and how to do it the right way. There is a talk at parleys where Jasper Potts and Jonathan Giles talk about creating a custom UI control. I wish I had seen this earlier.
1. Learn about the JavaFX seperation of concerns pattern
Like the most UIs, JavaFX has also a seperation of concerns pattern.
2. Refactor the MiniIconButton
So I have to break my 1-class component into 3 classes follow the API.
2.1 Control
The easiest one ist the Control. This is the model of the component and the part a developer is using in his code, like Button, TextField, Label, … I have to clean my class from all the code that has nothing to do with property handling. For each property a developer should be able to work with later on, I have to add a member variable XY based on
|
|
and add a getXY()-method a setXY()-method and a XY xYProperty()-method.
This results in the following code:
|
|
The MiniIconButton extends Button as it shares all the functionality with Button and just adds some new stuff.
2.2 CSS
But where is the part, where the skin is bound to the Control? That happens in the CSS! The Control has to override the
|
|
This stylesheet is responsible for the look and the connection to a skinning class. It is very important that the control sets a unique styleClass.
|
|
This styleClass is used to declare the connection.
|
|
2.3 Skin
In the MiniIconButtonSkin class happens all the UI stuff. Create internal components, draw all the things. The MiniIconButton extends Button, so the MiniIconButtonSkin extends ButtonSkin. Most of the work is done in this class and I benefit from extending it.
All the Skin classes extend StackPane. In the last version of the MiniIconAnimationButton, I have extended the StackPane in my button. That makes the refactoring easy. The code for adding the mini-icon to the stackpane is already there. It was just in the wrong class.
|
|
And these are the implementations of the methods.
|
|
Another change to the first version are the listeners added to properties. Without that, the button can never change after creation (except for the inheritat properties).
|
|
These possible changes are the reason why I decided to have the two animations as members in the skinning class. I need the possibility to start, stop and change them as a reaction to a property change. They could have been created lazy, but this is for the next version.
|
|
2.4 Behavior
The behavior classes are responsible for key and mouse handling. The MiniIconButton has no additional functionality and so no extra behavior class is needed.
2.5 Example
In the next Ensemble of the JFXtras Project, there is an example page for the MiniIconButton.
|
|
3. Demo
4. Sources
The Sources are part of the JFXtras Project.