Migrating climbing course website to Angular
Posted on March 18, 2017
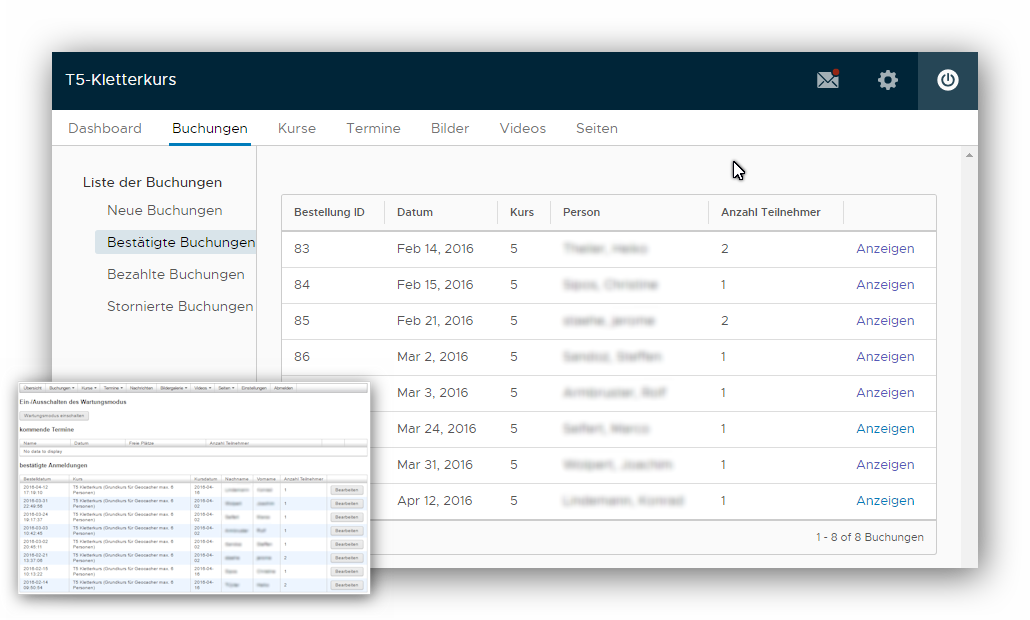
Introducing Angular 2 as Backend SPA for a climbing course website.
As my company decides to evaluate Angular 2 as our new framework to build our web application, I thought it would be a good experience to migrate one of my own projects to Angular 2.
1. T5 climbing course
Geocaching is a kind of sport, where people can search for little containers with the help of GPS. Some of those containers are only reachable with professional climbing stuff. On this site, people can order a workshop for professional climbing, taught by industrial climbers.
2. Old version
The old version is a classic CodeIgniter request-response application. On the client side the YUI-framework of YAHOO was used to build an interactive application.
On the serverside CodeIgniter is used with classic views.
2.1 Screenshots
The following screenshots show some pages of the application.
3. New version
3.1 REST
To allow Angular 2 to get the data from the current backend, I had to support REST. I decided to completely rewrite the backend to avoid using the old request-response backend. The CodeIgniter Rest Server helps serving REST with CodeIgniter.
|
|
|
|
3.2 Angular 2
Angular 2 with Angular CLI is a real productivity boost. Within a few days I managed to create a complete new backend for the site. Angular is still under heavy development and I haven’t explored all of the nice gimmicks the framework offers.
These are just a few points I love about Angular 2
- Typescript (and not Javascript)
- clean separation of concerns with modules, components, services, …
- dependency injection
- testing support
- support for SASS
- Routing
- Binding
3.3 Clarity Design System
There are a lot of UI component libraries and I tried the most of them. The clear look and the production ready state were the main points to chose the Clarity Design System provided by vmware.
The following points helped with the decision:
- DataGrid as the most important component in the application.
- Forms (Number-Field, Date-Field, …)
- application layout concept with navigation, alerts, …
3.4 Screenshots