Kotlin and TornadoFX
Posted on October 19, 2016
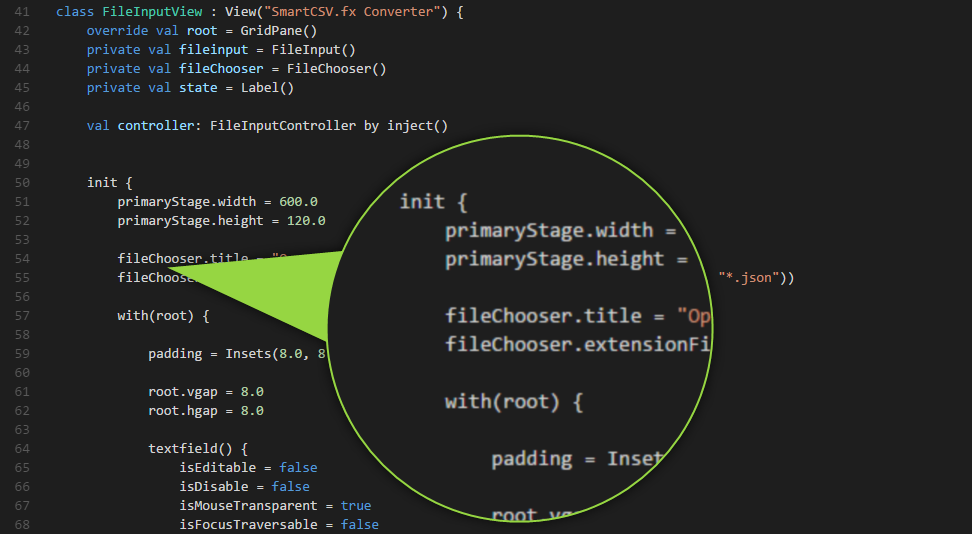
Converter for the old validation configuration written in Kotlin.
As I have changed the JSON for configure the SmartCSV.fx from my own Schema to JSON Table Schema, I had to provide a converter for existing configurations.
I wanted to write less code than I typically do in Java and learn something new. Therefor I started a new project in Kotlin a programming language invented by JetBrains, the people behind the excellent IntelliJ IDEA IDE.
To make my life even easier, I use klaxon a JSON parser for Kotlin, which allows a very simple way to access JSON artefacts.
|
|
|
|
As you can see, it is pretty straight forward to use klaxon. I just need the ? as the value may be null.
|
|
|
|
In my JSON config file, not all columns had to be defined. Only the ones with validation rules. That is the reason, why not each column has to be in the map and the column may be null.
|
|
When the column exist, just grab all possible values. In case of the boolean value I am using a kotlin construct ?: to provide a default value if the method returns null.
To step further into the code, just have a look at Converter.kt
TornadoFX
I wanted a simple JavaFX UI for the converter, as a command line call is not as comfortable as a button click. TornadoFX is a lightweight JavaFX framework for Kotlin, which allows you to define a JavaFX app in a few lines. Edvin Syse has done a great job, by providing an easy DSL to define a JavaFX UI.
|
|
This is more or less the complete definition of the UI including action handling for the buttons. While reading the code, you know exactly what this will look like!
For the complete source code, have a look at ConverterFX.kt. And that’s all. Two small Kotlin files! One for converting the JSON and one for the UI. Had so much fun and I am sure it could be done a lot better than I did!